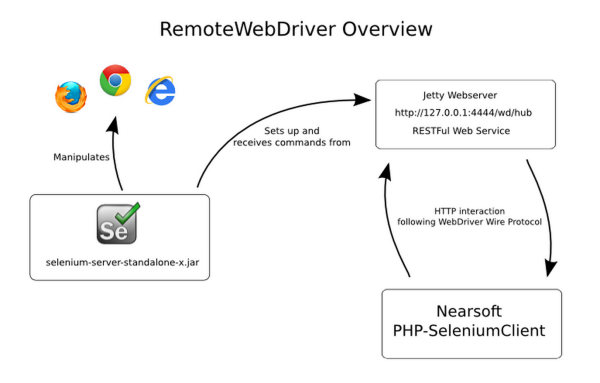
通过composer安装php-webdriver:
apt install php7.4-cli php-curl php-zip
curl -sS https://getcomposer.org/installer | php --install-dir=/usr/bin/
php composer.phar require php-webdriver/webdriver
安装java环境和selenium server:
apt install openjdk-14-jre
wget https://selenium-release.storage.googleapis.com/3.141/selenium-server-standalone-3.141.59.jar
java -jar selenium-server-standalone-3.141.59.jar
安装firefox/chrome浏览器和相应的webdirver:
wget https://dl.google.com/linux/direct/google-chrome-stable_current_amd64.deb
apt install ./google-chrome-stable_current_amd64.deb
wget https://chromedriver.storage.googleapis.com/88.0.4324.96/chromedriver_linux64.zip
unzip chromedriver_linux64.zip
mv chromedriver /usr/bin/
apt install firefox
wget https://github.com/mozilla/geckodriver/releases/download/v0.29.0/geckodriver-v0.29.0-linux64.tar.gz
tar zxf geckodriver-v0.29.0-linux64.tar.gz
mv geckodriver /usr/bin/
启动浏览器需X环境支持,可使用XVNC或X Window
可以使用Firefox扩展Selenium IDE: PHP Formatters录制脚本。
selenium chrome使用:
<?php
require_once('vendor/autoload.php');
use Facebook\WebDriver\Remote\DesiredCapabilities;
use Facebook\WebDriver\Remote\RemoteWebDriver;
use Facebook\WebDriver\Chrome\ChromeOptions;
$host = 'http://localhost:4444/wd/hub';
$options = new ChromeOptions();
$options->addArguments(array(
'--no-sandbox',
'--headless',
'--start-maximized',
'--user-data-dir=/tmp/chrome-user-data-dir',
'--profile-directory=/tmp/chrome-profile-dir',
'--user-agent=Mozilla/5.0 (X11; Linux x86_64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/88.0.4324.182 Safari/537.36'
));
$caps = DesiredCapabilities::chrome();
$caps->setCapability(ChromeOptions::CAPABILITY, $options);
$driver = RemoteWebDriver::create($host, $caps);
//default
//$driver = RemoteWebDriver::create($host, DesiredCapabilities::chrome());
//$driver->manage()->window()->maximize();
$driver->get('https://www.haiyun.me/');
var_dump($driver->getTitle());
$driver->quit();
selenium firefox使用:
<?php
namespace Facebook\WebDriver;
require 'vendor/autoload.php';
use Facebook\WebDriver\Remote\RemoteWebDriver;
use Facebook\WebDriver\Remote\DesiredCapabilities;
use Facebook\WebDriver\Firefox\FirefoxProfile;
use Facebook\WebDriver\Firefox\FirefoxDriver;
$host = 'http://localhost:4444/wd/hub';
$profile = new FirefoxProfile();
$profile->setPreference('browser.startup.homepage', 'https://github.com/facebook/php-webdriver/');
$profile->setPreference("general.useragent.override", "Mozilla/5.0");
//$profile->addExtension('./vimperator-3.8.2-fx.xpi');
$caps = DesiredCapabilities::firefox();
$caps->setCapability(FirefoxDriver::PROFILE, $profile);
$caps->setCapability('moz:firefoxOptions', ['args' => ['-headless']]);
$caps->setCapability('moz:firefoxOptions', ['args' => ["-profile", "/tmp/firefox_profile"]]);
$driver = RemoteWebDriver::create($host, $caps);
//default
//$driver = RemoteWebDriver::create($host, DesiredCapabilities::firefox());
$driver->manage()->window()->maximize();
$driver->get('https://www.haiyun.me/');
var_dump($driver->getTitle());
$driver->quit();
文档:
https://github.com/php-webdriver/php-webdriver/wiki
https://php-webdriver.github.io/php-webdriver/